Implementation of SonarQube in a Spring Boot application
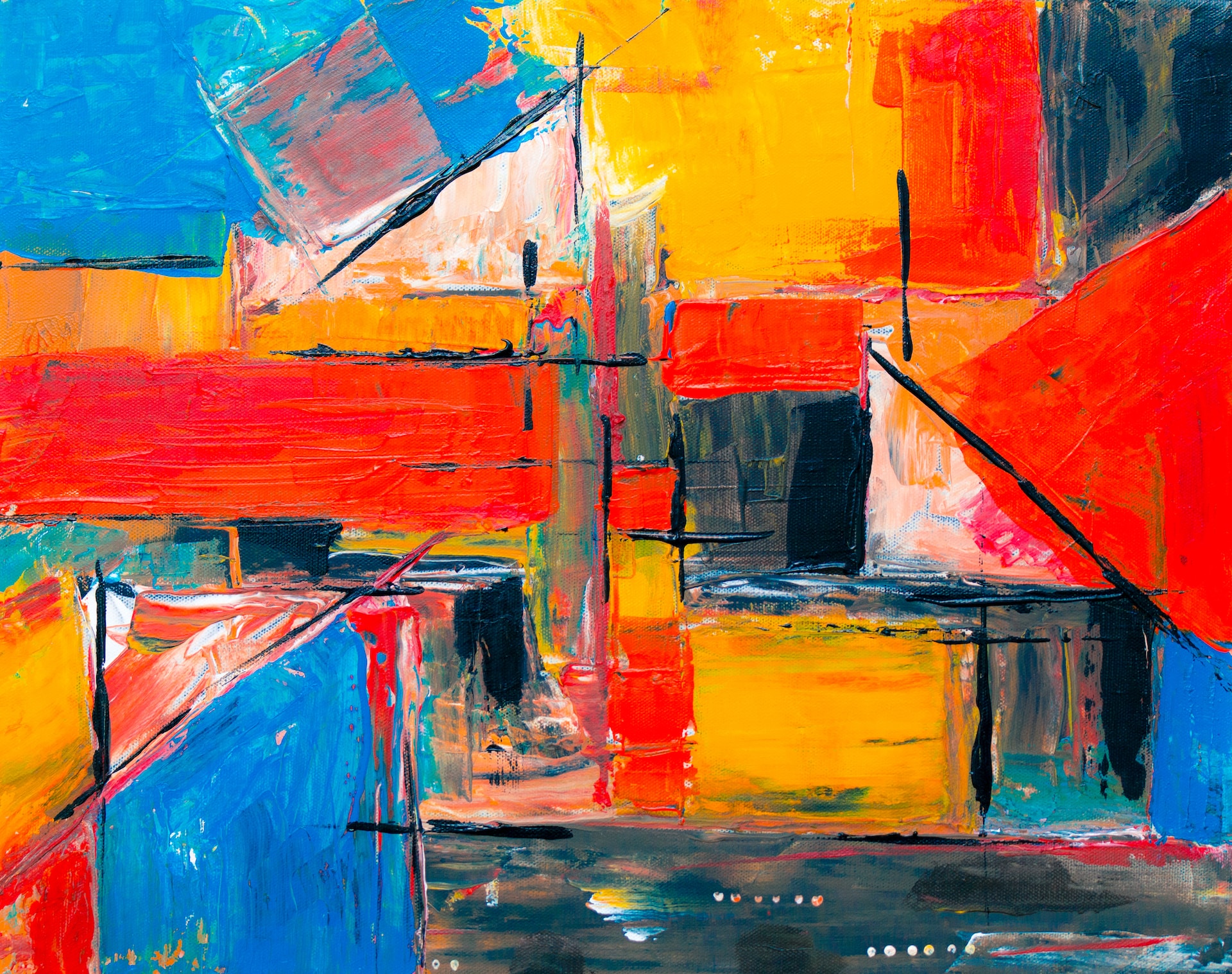
Implementation of SonarQube in a Spring Boot application.
What is SonarQube?
SonarQube is an open-source platform for static code analysis that assists developers and teams in monitoring and improving the quality of their source code. The main objectives of SonarQube include identifying code smells, bugs, and security vulnerabilities, as well as monitoring code quality over time.
Create docker-compose.yml
docker-compose.yml
|
|
SonarQube Service
container_name
: Specifies the name of the Docker container for the SonarQube service.image
: Specifies the Docker image to be used for the SonarQube service (in this case, “sonarqube”).depends_on
: Defines the dependency of the SonarQube service on another service (sonarqube-database) to ensure that the database is started before SonarQube.environment
: Sets environment variables for the SonarQube application, including JDBC connection data to connect to the PostgreSQL database.volumes
: Connects Docker volumes to specific directories in the SonarQube container to persistently store data.ports
: Makes the SonarQube service accessible on the host through port 9000.
SonarQube Database Service
container_name
: Specifies the name of the Docker container for the SonarQube database.image
: Specifies the Docker image for the PostgreSQL database.environment
: Sets environment variables for configuring the PostgreSQL database.volumes
: Links Docker volumes to directories in the database container to persistently store data.ports
: Publishes the database service on port 5432 on the host.
Volumes
Defines various Docker volumes used for the persistent data of the SonarQube service and the database. These volumes allow maintaining data between container instances even when the containers are restarted.
Start docker-compose
|
|
Stop docker-compose
|
|
Integration of SonarQube into a Spring Boot Gradle project
The integration of SonarQube into a Spring Boot Gradle project is done through the build.gradle
file.
Alternatively, this can be done through the sonar-project.properties
file in the root directory of the project.
Integration of the SonarQube plugin
|
|
Setting the SonarQube parameters
|
|
Explanation of SonarQube parameters
sonar.host.url
: The URL of SonarQube to which the analysis results should be sent.sonar.token
: The project key used for authentication and authorization. Here, the token for your specific project should be provided.sonar.projectKey
: The unique key that identifies the project in SonarQube.sonar.projectName
: The name of the project as displayed in SonarQube.sonar.sources
: The directory containing the source code files for analysis.sonar.java.source
: The Java version used for source code analysis.sonar.gradle.skipCompile
: Whether compilation should be skipped during SonarQube analysis.sonar.test.exclusions
: The directories or file paths to be excluded from test analysis.sonar.exclusions
: The directories or file paths to be excluded from general code analysis.sonar.coverage.exclusions
: File paths to be excluded from code coverage analysis.sonar.scm.disabled
: Whether the integration of Software Configuration Management (SCM) should be disabled during analysis.encoding
undcharSet
: Setting the character encoding for code analysis.
After the analysis has been performed, the project should be accessible at http://localhost:9000.
The default login credentials for SonarQube are admin
(username) and admin
(password).